Introduction
Update individual records using Entity Framework is very easy but what about a bulk update?In one of my article, I have shown you how to insert multiple rows to a database using asp.net MVC. but recently I came across a scenario when I need to implement a bulk update operation through a Web interface. Here In this article, I have explained how to update multiple rows at once using entity framework in asp.net MVC application.
If you have asp.net webforms project and using stored procedure, please visit how to update bulk data (multiple rows) to a SQL server database in asp.net webform. and for insert multiple rows at once in asp.net webforms visit how to update bulk data (multiple rows) to a SQL Server database using ASP.NET
Prerequisite
I used followings:- .Net framework 4.0
- Entity Framework
- Jquery
- Sql Server 2008
Steps
Just follow the steps and get result easily.Step-1: Create New Project
First we need to create a project.Go to Menu File > New > Project > select asp.net mvc4 web application > Entry Application Name > Click OK.
Step-2: Add a Database.
Go to Solution Explorer > Right Click on App_Data folder > Add > New item > Select SQL Server Database Under Data > Enter Database name > Add.

Step-3: Add Entity Data Model.
Go to Solution Explorer > Right Click on Project Name from Solution Explorer folder > Add > New item > Select ADO.net Entity Data Model under data > Enter model name > Add.
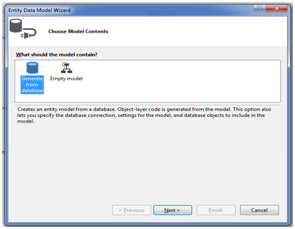
Here we need to modify contact.cs file

namespace UpdateMultiRecord { using System; using System.Collections.Generic; using System.ComponentModel.DataAnnotations; public partial class Contact { [Required] public int ContactID { get; set; } [Required] public string ContactPerson { get; set; } [Required] public string Contactno { get; set; } public string EmailID { get; set; } } }
Step-4: Implement Action for Get & Post Method.
Here I am using Home controller index action.Get Action
[HttpGet] public ActionResult Index() { List<Contact> model = new List<Contact>(); using (MyDatabaseEntities dc = new MyDatabaseEntities()) { model = dc.Contacts.ToList(); } return View(model); }Post Action
[HttpPost] public ActionResult Index(List<Contact> list) { if (ModelState.IsValid) { using (MyDatabaseEntities dc = new MyDatabaseEntities()) { foreach (var i in list) { var c = dc.Contacts.Where(a =>a.ContactID.Equals(i.ContactID)).FirstOrDefault(); if (c != null) { c.ContactPerson = i.ContactPerson; c.Contactno = i.Contactno; c.EmailID = i.EmailID; } } dc.SaveChanges(); } ViewBag.Message = "Successfully Updated."; return View(list); } else { ViewBag.Message = "Failed ! Please try again."; return View(list); } }
Step-5: Create View for Update Multiple Row.
Our View Index.cshtml@model List<UpdateMultiRecord.Contact> @{ ViewBag.Title = "Update multiple row at once Using MVC 4 and EF "; } @using (@Html.BeginForm("Index","Home", FormMethod.Post)) { <table> <tr> <th></th> <th>Contact Person</th> <th>Contact No</th> <th>Email ID</th> </tr> @for (int i = 0; i < Model.Count; i++) { <tr> <td> @Html.HiddenFor(model => model[i].ContactID)</td> <td>@Html.EditorFor(model => model[i].ContactPerson)</td> <td>@Html.EditorFor(model => model[i].Contactno)</td> <td>@Html.EditorFor(model => model[i].EmailID)</td> </tr> } </table> <p><input type="submit" value="Save" /></p> <p style="color:green; font-size:12px;"> @ViewBag.Message </p> } @section Scripts{ @Scripts.Render("~/bundles/jqueryval") }
Here
@Scripts.Render("~/bundles/jqueryval")this will enable client side validation.
Step-6: Run Application.
Edit Contact Details and Click Save button.